Time ago snippet guide
This quick guide demonstrates how to create a JavaScript snippet that converts date times into hours, days, minutes, and seconds from the current moment.
Steps
-
Create a Budibase internal table this should include some form of date (In my case I will theme this around a blog)
-
Add data to your table
-
Go to the design screen and add a Card block
-
Fill in the relevant bindings for Title, description etc
-
Go to the subtitle bindable area.
-
Switch the tab from text to javaScript
-
Click on the
</>
icon and create a new snippet calledtimeago
-
Paste the below code into it and click save
return function(pastTimeString) {
const pastTime = new Date(pastTimeString);
const now = new Date();
const delta = now - pastTime;
const days = Math.floor(delta / (1000 * 60 * 60 * 24));
const hours = Math.floor((delta % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((delta % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((delta % (1000 * 60)) / 1000);
const timeStrings = [];
if (days > 0) {
timeStrings.push(`${days} days`);
}
if (hours > 0) {
timeStrings.push(`${hours} hours`);
}
if (minutes > 0) {
timeStrings.push(`${minutes} minutes`);
}
if (seconds > 0) {
timeStrings.push(`${seconds} seconds`);
}
if (timeStrings.length === 0) {
return "just now";
} else if (timeStrings.length === 1) {
return timeStrings[0];
} else {
return timeStrings.slice(0, -1).join(', ') + ', and ' + timeStrings.slice(-1) + ' ago';
}
}
- Click the snippet and add the date binding you wish to use inside its brackets
- Then click save
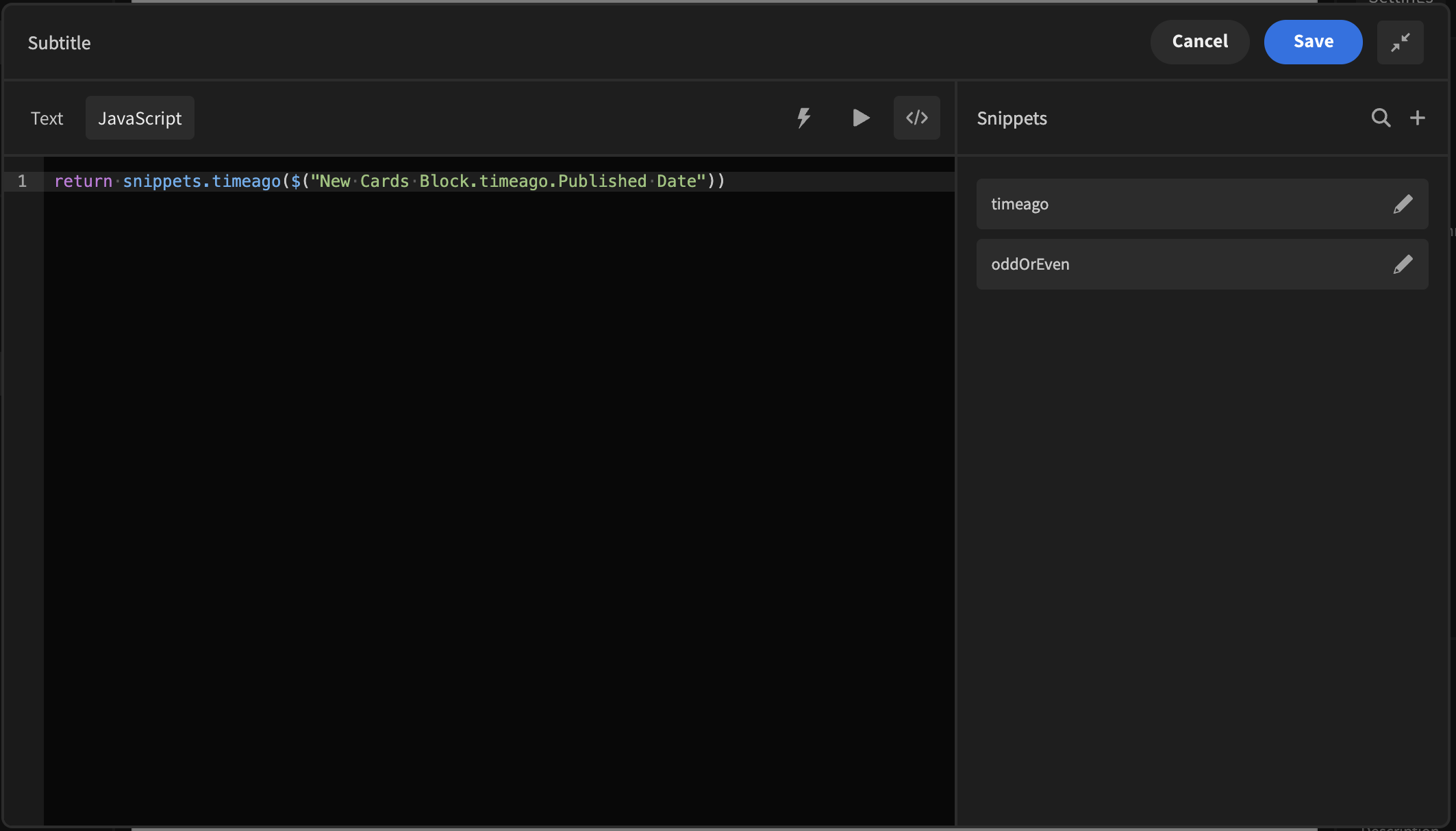
The end result!
Updated 10 months ago