JS Scripting
Adding a JavaScript code block step to your automations
While Bindings in automations support JavaScript, you can also add a step to execute a JavaScript code block.
Commonly this action is used to compare and aggregate data from multiple Datasources.
Adding a JavaScript code block
- After adding an automation Trigger, click the
+
icon and selectJS Scripting
. ClickSave
.
- Click the
Edit Code
button to open the code editor modal.
Make sure to return a value at the end of your script!
- Expand the BINDINGS section to see the available bindings that can be used in your script. The path indicates how to reference these variables.
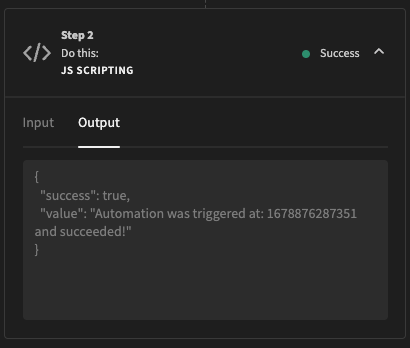
Example output
Tutorial: Linking an API response to table rows
In this tutorial we will look at using a JS Scripting block to link people from an API response to people with the same name in a Budibase table.
- Add a REST Query. This example will pull back the Top 100 Birders for a given date. Be sure to
Save
the query afterSend
.
- We will also add a Budibase DB table for the members of our mock bird club, which will include some additional information not provided by the API, such as Twitter Username.
- In the Automate tab, add an automation. This automation will fetch data from both sources and send a summary Email. I will use an App action trigger, but any other trigger could be used.
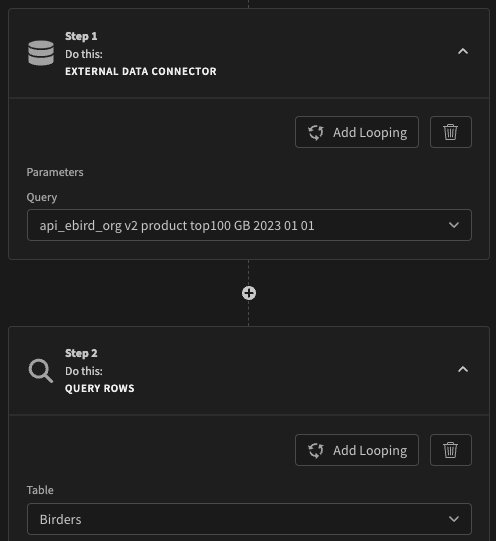
Fetch the Top 100 birders from the API, and then all our club members
- Add a
JS Scripting
block, andEdit Code
. Enter the following:
/*
* Compare and combine the local club members that match, by name, with the Top 100 birders from the API.
*/
const top100 = steps[1].response;
const clubMembers = steps[2].rows;
let topClubMembers = top100.filter(row => clubMembers.map(tableRow => tableRow.Name).includes(row.userDisplayName));
return topClubMembers.map(member => ({
...member,
...clubMembers.find(tableRow => tableRow.Name === member.userDisplayName)
}));
- Finally add the
Send Email
step. Fill in the details and format the HTML Contents as desired.
let listItems = ""
for (let member of $("steps.3.value")) {
listItems += `<li>${member.Name} <b>@${member["Twitter Username"]}</b>
<br />Spotted ${member.numSpecies} species!</li><br/>`
}
return `Congrats to our top bird watchers - give them a shout out on Twitter!
<p><ol>${listItems}</ol></p>`
- Click
Finish and test automation
to see the result!
Updated 5 months ago