Update date field on change
For example automatically setting an end date when a start date is selected
Try it out!
Scenario
You're building an app and you want to set a project length in days, and then dynamically calculate start and end dates.
★★★☆☆
Steps
Add component
: Form- In the Settings Panel set Schema to 'Jobs'
Add component
: Container- In the Settings Panel set Direction to 'Row'
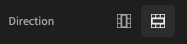
Add component
: Number Field- Manually enter 'Direction' into the Field name and set Label to 'Days of work'
- Set Default value to 7
Configure validation
:Add Rule
: Min value 'Value' 1 Cannot be less than one day!Add Rule
: Max value 'Value' 365 Cannot be more than 365 days!Save
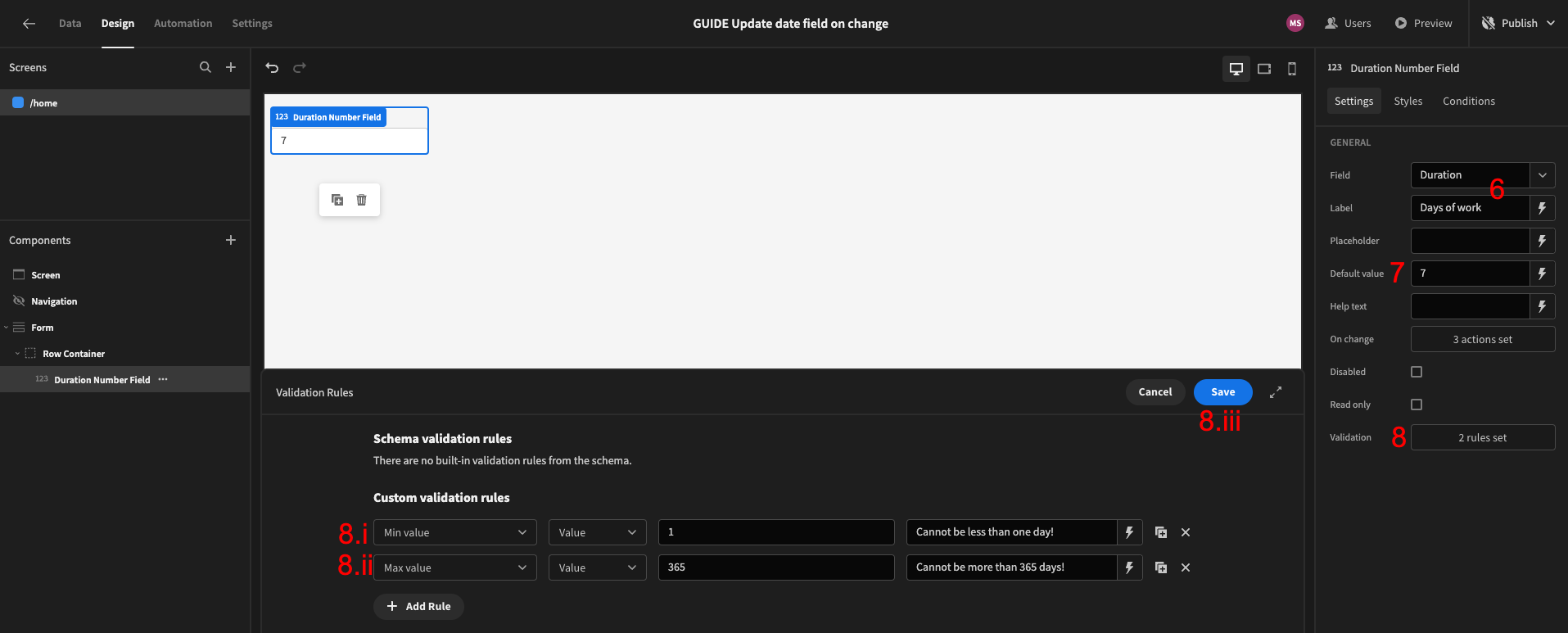
Add component
: Date Picker- In the Settings Panel select 'Works Start' from the Field dropdown
- Set Label to 'Works Start'
- Untick Show time
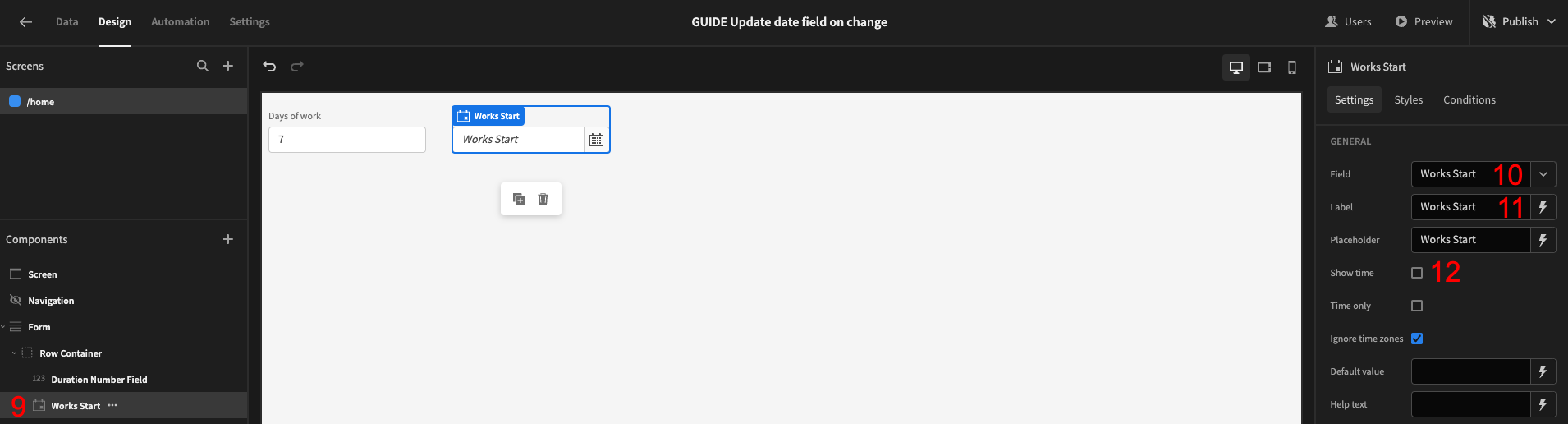
- Duplicate the Date Picker
- Replace 'Start' with 'End' in the Field and Label settings
Time to make the field values dynamically update!
-
In the Settings Panel under On change:
Define actions
-
Validate Form - make sure to select the form in the dropdown
-
Update Field Value
- select the Form from the dropdown
- select 'Set value' as the Type
- select 'Works Start' as the Field
- click the lightning bolt icon, and click on the
JavaScript
tab. Provide the following:
//Source: https://stackoverflow.com/questions/563406/how-to-add-days-to-date Date.prototype.subtractDays = function(days) { var date = new Date(this.valueOf()); date.setDate(date.getDate() - days); return date; } return new Date($("Field Value")).subtractDays($("Jobs Form.Fields.Duration"));
-
The Validate Form action will prevent the Update Field Value from firing if there's any validation errors.
The special
$("Field Value")
binding is used to indicate that the On change value should be used, and not the value before the change.Also note that you may need to replace the
$("Jobs Form.Fields.Duration")
binding to match your form name.
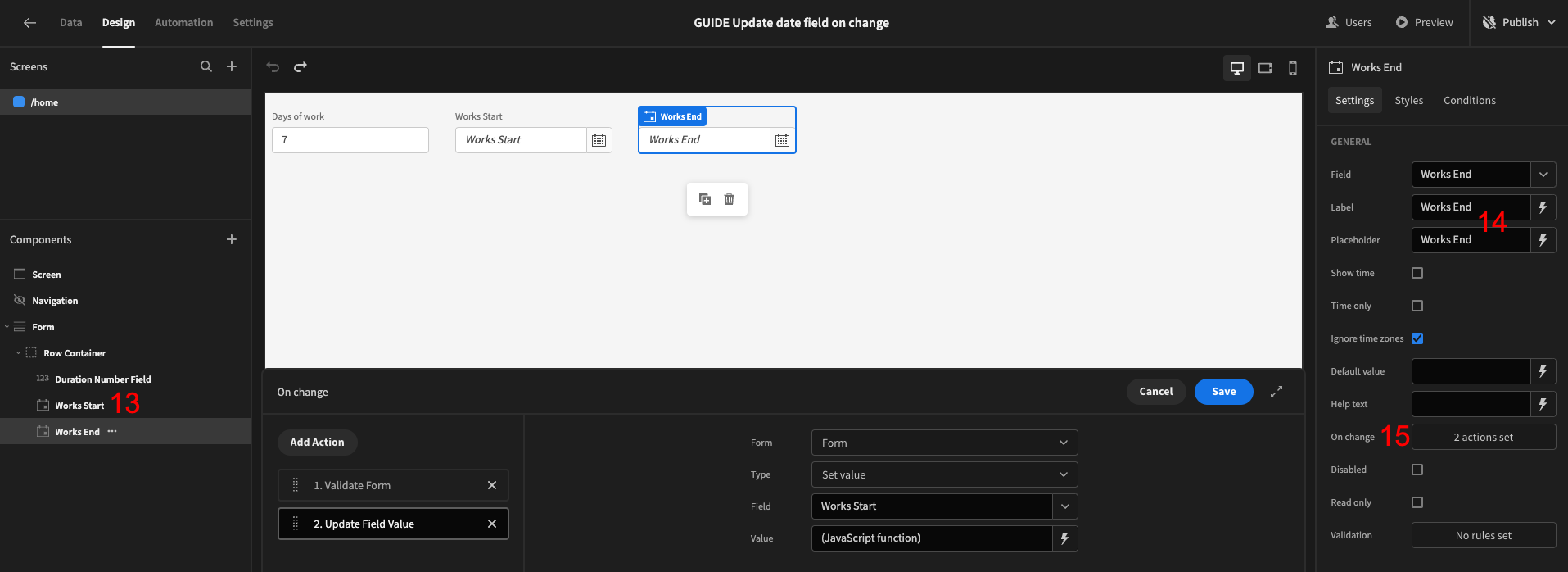
-
Select the Works Start Date Picker. Under On change:
Define actions
- Validate Form - make sure to select the form in the dropdown
- Update Field Value - similar to before, but this time we are setting the value of the 'Works End' field, and will add days instead of subtracting:
//Source: https://stackoverflow.com/questions/563406/how-to-add-days-to-date Date.prototype.addDays = function(days) { var date = new Date(this.valueOf()); date.setDate(date.getDate() + days); return date; } return new Date($("Field Value")).addDays($("Jobs Form.Fields.Duration"));
-
Finally click on the Number Field. Under On change:
Define actions
-
Validate Form - make sure to select the form in the dropdown
-
Continue if / Stop if - 'Stop if'
{{ Jobs Form.Fields.Works Start }}
'Equals' "" -
Update Field Value:
- select the Form from the dropdown
- select 'Set value' as the Type
- select 'Works End' as the Field
- click the lightning bolt icon, and click on the
JavaScript
tab. Provide the following:
//Source: https://stackoverflow.com/questions/563406/how-to-add-days-to-date Date.prototype.addDays = function(days) { var date = new Date(this.valueOf()); date.setDate(date.getDate() + days); return date; } return new Date($("Form.Fields.Works Start")).addDays($("Field Value"));
-
In this case we first check that the number of days is a valid number.
Next we use the Stop if action to make sure a 'Works Start' date has been chosen, because we need to know the start date in order to calculate the end date.
App export
Downloads may take a few seconds.
Updated 5 months ago